Computer Networking - TCP/UDP
Internet Protocol Stack
- Application: supporting network applications - FTP, SMTP, HTTP
- Transport: process-process data transfer - TCP, UDP
- Network: routing of datagrams from source to destination - IP, routing protocols
- Link: data transfer between neighboring network elements - Ethernet, 802.111 (WiFi), PPP
- Physical: bits “on the wire”
What Transport Service Does An App Need?
- Data integrity
-Some apps (e.g., File transfer, web transactions) require 100% reliable data transfer
-Other apps (e.g., Audio) can tolerate some loss - Timing
-Some apps (e.g., Internet telephony, interactive games) require low delay to be “effective” - Throughput
-Some apps (e.g., multimedia) require minimum amount of throughput to be “effective”
-Other apps (“elastic apps”) make use of whatever throughput they get - Security
-Encryption, data confidentiality, …
Internet Transport Protocols Services
- TCP service:
Reliable transport: between sending and receiving process.
Flow control:sender won’t overwhelm receiver.
Congestion control: throttle sender when network overloaded.
Does not provide: timing, minimum throughput guarantee, security.
Connection-oriented: setup required between client and server processes.
- UDP service:
Unreliable data transfer: between sending and receiving process.
Does not provide: reliability, flow control, congestion control, timing, throughput guarantee, security, or connection setup.
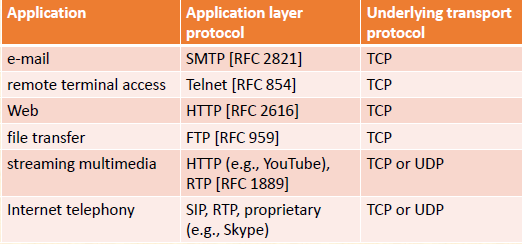
Socket Programming
- Goal: learn how to build client/server applications that communicate using sockets
- Socket: door between application process and end-end-transport protocol
Two socket types for two transport services:
- UDP: unreliable datagram
- TCP: reliable, byte stream-oriented
Application Example:
- Client reads a line of characters (data) from its keyboard and sends data to server
- Server receives the data and converts characters to uppercase
- Server sends modified data to client
- Client receives modified data and displays line on its screen
Socket Programming With UDP
- UDP: no “connection”between client & server
No handshaking before sending data.
Sender explicitly attaches IP destination address and port # to each packet.
Receiver extracts sender IP address and port# from received packet.
- UDP: transmitted data may be lost or received out-of-order
- Application viewpoint: UDP provides unreliabletransfer of groups of bytes (“datagrams”) between client and server
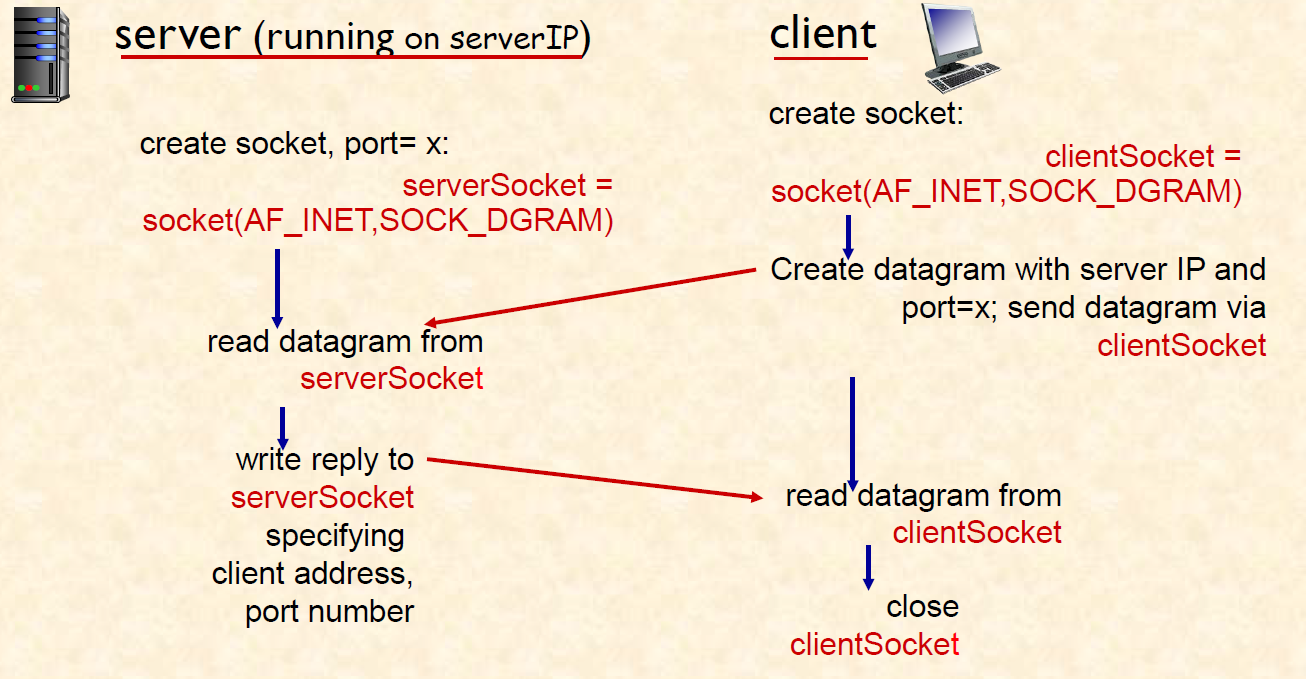
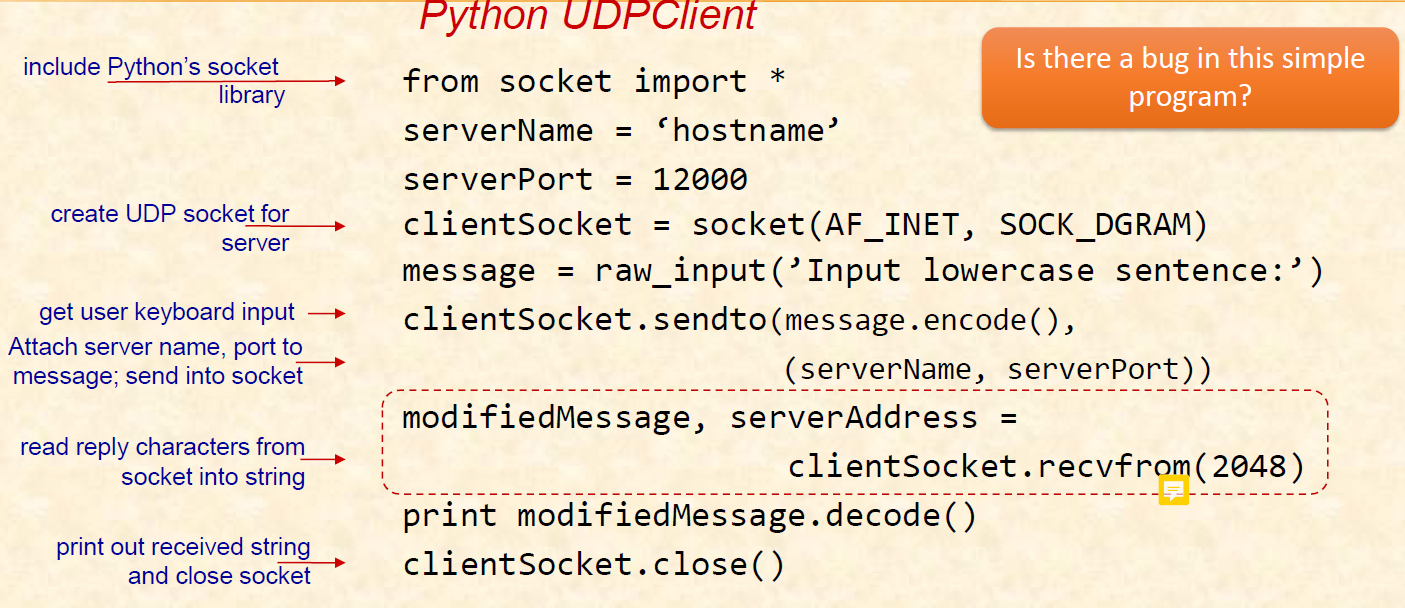
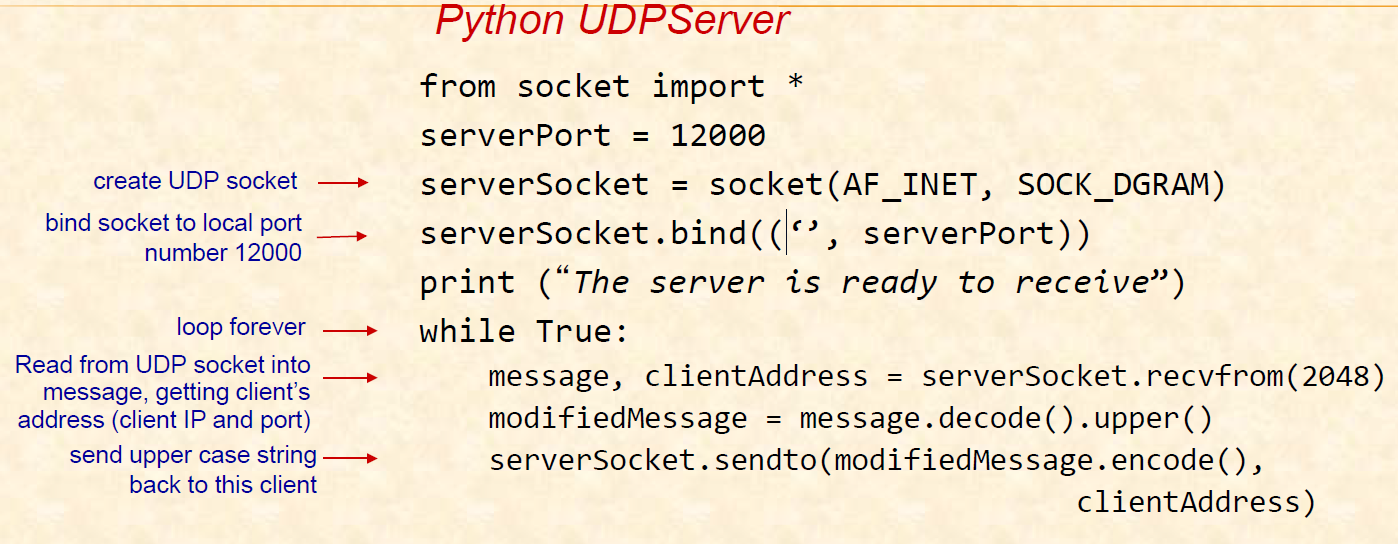
Client/Server Socket Interaction: TCP
- Client must contact server
Server Process Must First Be Running.
Server Must Have Created Socket (Door) That Welcomes Client’s Contact.
- Client contacts server by:
Creating TCP socket, specifying IP address, port number of server process.
When client creates socket: client TCP establishes connection to server TCP.
- When contacted by client, server TCP creates new socketfor server process to communicate with that particular client
Allows server to talk with multiple clients.
Source port numbers used to distinguish clients (more in Chap 3).
- Application viewpoint: TCP provides reliable, in-order Byte-stream transfer (“pipe”) between client and server
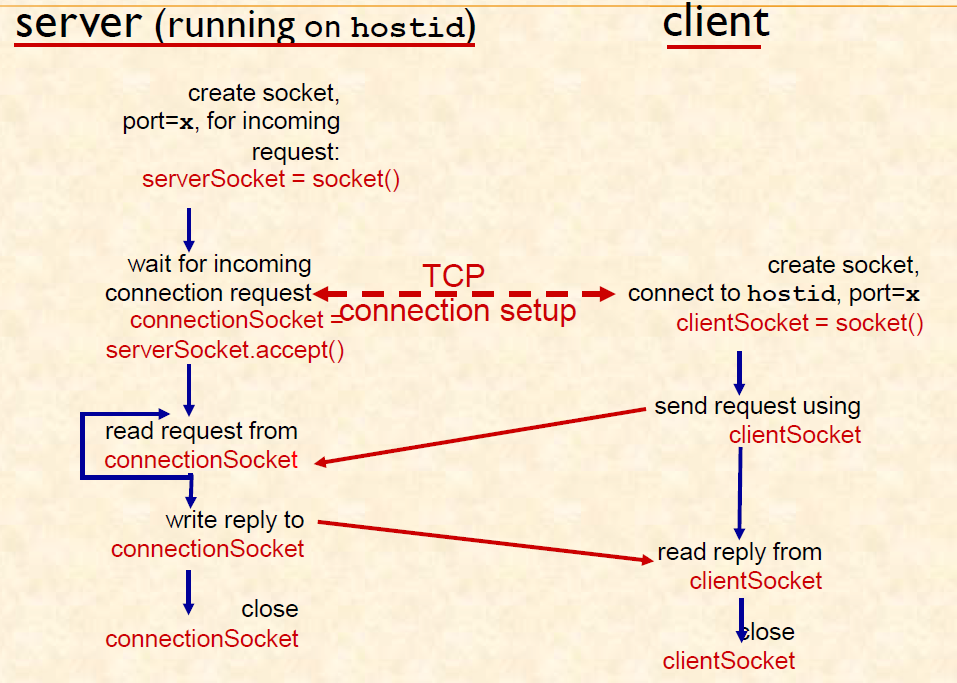
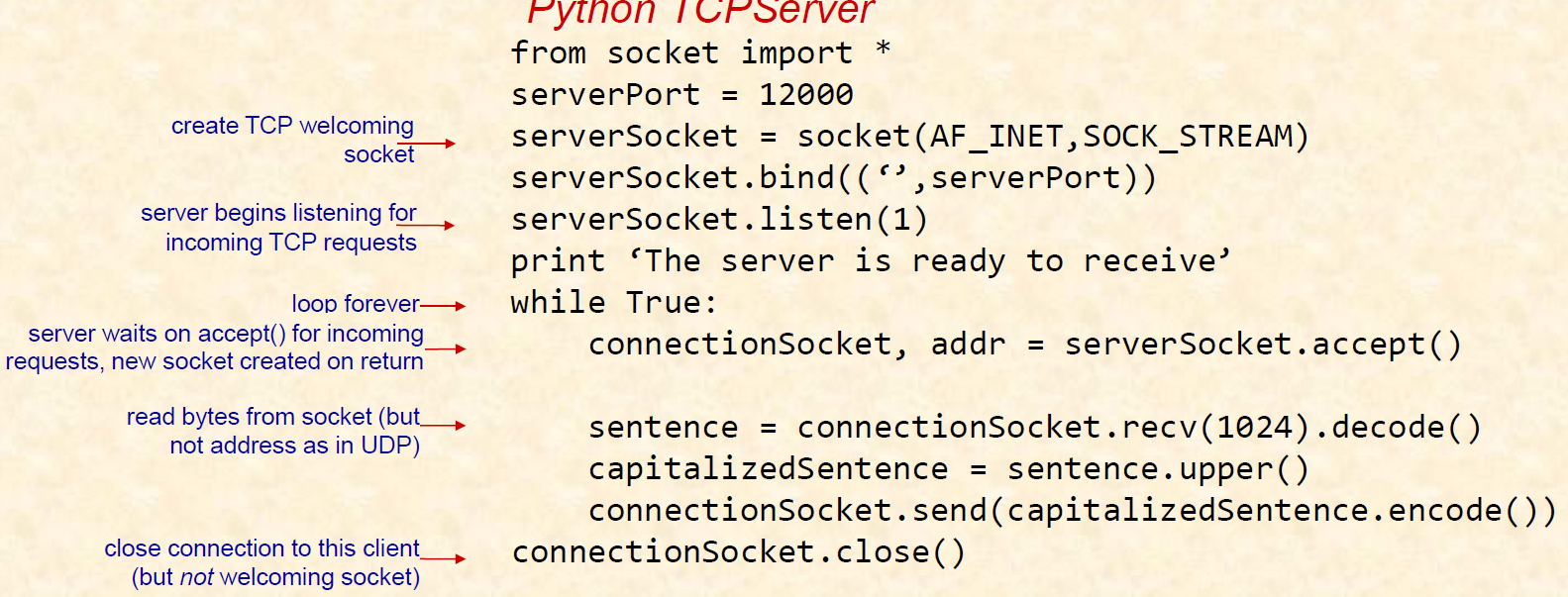
a. TCP Client/Server (2 Programs)
b. Reliable UDP Client/Server (2 programs)
c. Unreliable UDP Client/Server (2 Programs)
A good reference for Python socket programming : https://docs.python.org/3/howto/sockets.html
The Server - a reliable environment
The server performs the Operation Code (OC) requested on the two integer numbers it receives from the sender and returns the result. The format of the returned result is “status-code numeric-result” (without quote), as explained below. More specifically the steps (or algorithm) performed by the server are:
- Open a TCP Socket:
- Initialize and bind a TCP socket to listen for incoming connections.
- Listen to the Socket:
- Put the socket into listening mode to accept connection requests.
- Accept Connection and Receive Request:
- Accept a new connection.
- Receive a request consisting of an Operation Code (OC) and two integer numbers.
- Validate Request:
- Check if OC is one of
+
,-
,*
,/
. - Ensure both operands are integers.
- Handle division by zero for
/
.
- Check if OC is one of
- Error Handling:
- For invalid OC, send status code
620
and result-1
. - For invalid operands or division by zero, send status code
630
and result-1
.
- For invalid OC, send status code
- Process Valid Request:
- If request is valid, perform the operation.
- Send back status code
200
and the result.
- Logging:
- Output
{received_line} -> {status_code} {result}
to standard output.
- Output
- Loop Back:
- Return to Step 2 for handling new requests.
- Shutdown:
- Respond to
^C
(Control-C) signal to gracefully stop the server.
- Respond to
The Client - a reliable environment
The client sends an Operation Code (OC), and the two numbers it has acquired from the user. OC can be: Addition (+), Subtraction (-), Multiplication (*), and Division (/)
To make the problem simple, your client sends two numbers that are integers.
The two numbers and the OC are read from a file which the user has prepared. The algorithm is specified as follows:
- Start and Read Input File:
- Start the client program, accepting a filename as a command-line argument.
- Read the file line by line, where each line contains an OC and two integers, separated by spaces.
- Display Input Request:
- For each line read, display
Input request: {input_line}
.
- For each line read, display
- Open a TCP Socket:
- Establish a TCP connection to the server.
- Send Request to Server:
- Send the line read from the file to the server.
- Receive Response:
- Wait for and receive the response from the server (status code and result).
- Process Response:
- If status code is
200
, displayThe result is: {result}
. - For other status codes, display
Error {status_code}: {description}
.
- If status code is
- Close Socket:
- Close the TCP connection.
- Repeat for 7 Lines:
- Repeat steps 2-6 for a total of 7 lines from the file.
- Terminate Program:
- Stop the client program.
The UDP Server - an unreliable environment
- Start and Read Command Line Parameters:
- On startup, read two parameters from the command line: the probability
p
of dropping a received datagram and a string for seeding a random number generator.
- On startup, read two parameters from the command line: the probability
- Seed Random Number Generator:
- Use
random.seed(sys.argv[2])
to seed the random number generator with the provided string. This ensures reproducibility of the random behavior.
- Use
- Open a UDP Socket:
- Initialize and bind a UDP socket for the server.
- Listen to the Socket:
- Wait for incoming UDP datagrams.
- Receive a Datagram:
- Receive a request in the form of a datagram.
- Simulate Datagram Drop:
- With probability
p
, determined byif random.random() <= p
, drop the request. - If dropped, print
{received_line} -> dropped
and return to Step 4.
- With probability
- Parse the Request:
- Extract the operation code (OC) and two integer numbers from the received datagram.
- Validate the Request:
- Check if OC is one of
+
,-
,*
,/
. - Ensure both operands are integers.
- Handle division by zero for
/
.
- Check if OC is one of
- Handle Invalid Requests:
- For an invalid OC, return status code
620
and result-1
. - For invalid operands or division by zero, return status code
630
and result-1
.
- For an invalid OC, return status code
- Process Valid Requests:
- If the request is valid, perform the operation.
- Return status code
200
and the operation result.
- Logging:
- Print
{received_line} -> {status_code} {result}
to standard output.
- Print
- Loop Back:
- Return to Step 4 for handling new datagrams.
- Shutdown Handling:
- The server should respond to
^C
(Control-C) for graceful termination.
- The server should respond to
The UDP Client - an unreliable environment
- Start and Read Input File:
- Start the client and read the name of a file passed as a command-line parameter.
- The file contains lines with three values: an Operation Code (OC) and two integer numbers, separated by spaces.
- Initialize Timer Value:
- Set
d = 0.1
seconds (initial timeout duration).
- Set
- Open a UDP Socket:
- Create and configure a UDP socket.
- Send Request to Server:
- Read a line from the file and send it to the server via the UDP socket.
- Start Timer and Wait for Reply:
- Start a timer for
d
seconds. - Wait for a reply from the server within this time frame.
- Start a timer for
- Handle Timer Expiry:
- If the timer expires before a reply is received:
- Double the timer value (
d = 2 * d
). - If
d > 2
seconds:- Raise an exception.
- Notify the user with “Request timed out: the server is DEAD”.
- Set status code to
300
(indicating server unavailability). - Proceed to Step 8 (turn off the timer).
- Otherwise, print “Request timed out: resending” and go back to Step 4.
- Double the timer value (
- If the timer expires before a reply is received:
- Receive Reply from Server:
- If a reply is received before timeout, receive the status code and result.
- Turn Off Timer:
- Stop the timer.
- Process Server Response:
- If the status code is
200
, display “Result is {result}”. - For other status codes, display “Error {status_code}: {description}”.
- If the status code is
- Close the Socket:
- Close the UDP socket.
- Repeat for Additional Requests:
- Repeat steps 2-10 six more times, processing a total of seven lines from the input file.
- Stop the Program:
- Terminate the client program.
Reference:Prof. Parviz Kermani